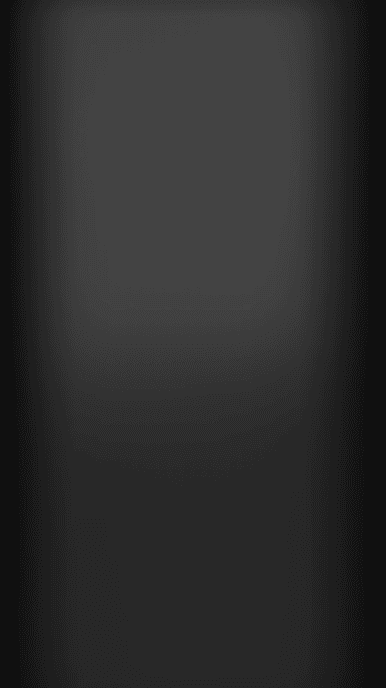
THE AUDIO MANAGER
VIDEO
When the pipeline is completed this is the kind of result we get shown in the video!
( Use headphones for best experience )
We will be going thorugh how I go from concept and design audio, how i set up the FMOD project.
We will go though how the AudioManager is set up in header file and cpp file.
After that we wil look at some functions the we will use outside in the gamecode.
And ofcourse we will look at some examples on how we utilize the AudioManager and its functions.
Lastly i will give some thought on what i feel i want to improve and why some things are set up like it is right now.
But I will also give some insight in some problems i had and how i solved them.
You can navigate the chapters using the dropdown in the top right!
OBS!
Keep in mind that TGA ( the school i am currently student at ) does not teach anything about audio programming, nor does anyone at our school in stockholm know how any audio API operates. so with this I am self tought.
And considering that Audio is nothing we get grades on and other things do, other areas takes priority. I can only iterate and improve the AudioManager when time approves.
( I have become the "Audio Caveman" all other programmers go to for help with FMOD )
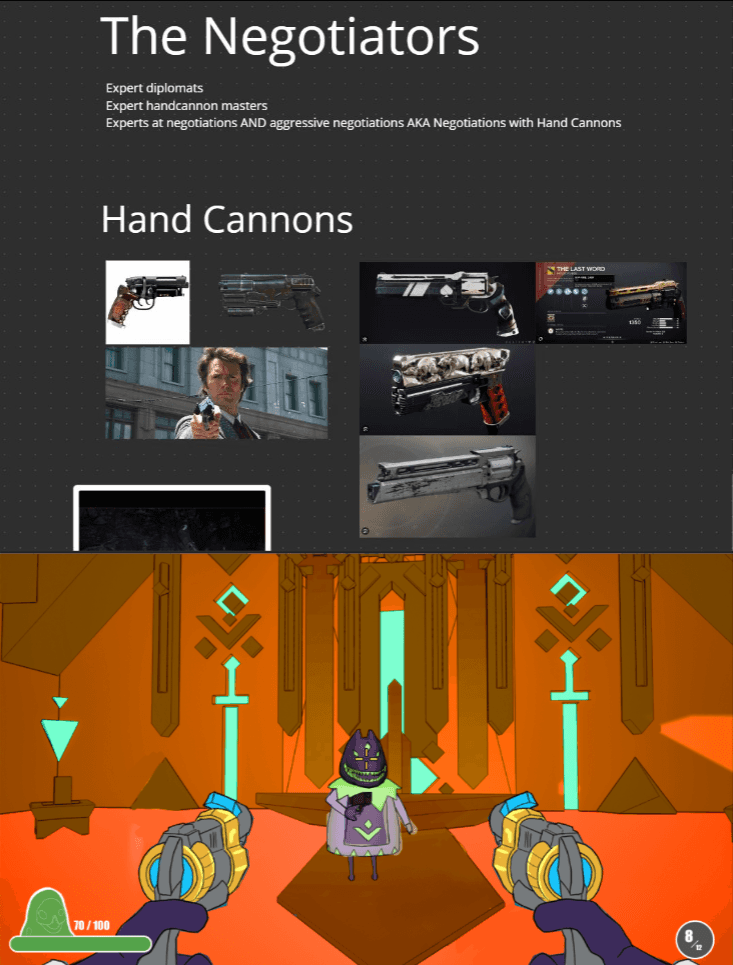
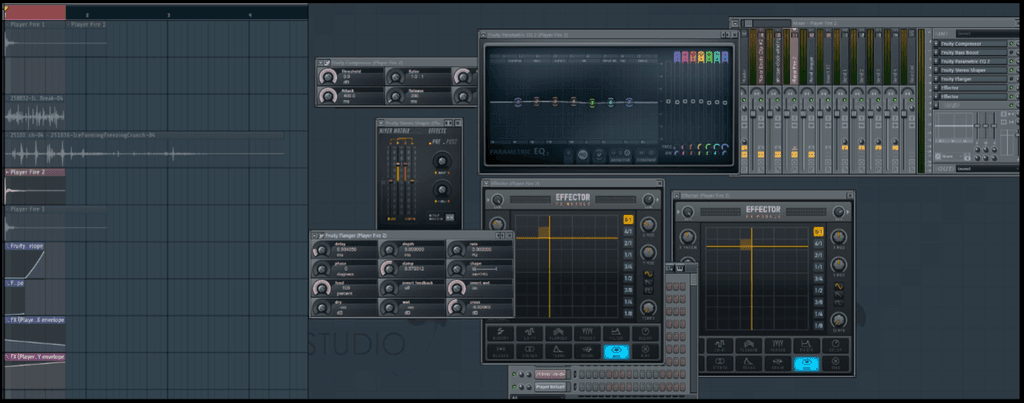
Fisrt I get the concept of what will have an audio sample. I get a decription of the feel we want to achieve, in this example I use the Hand Cannons, they ought to sound like handhelt space canons with alot of punch.
With the concept art I can more easily get the idea of the power level that is reasonable.
I start right away to mix the audio sample i found on Soundsnap.com. the audio sample is an M1 Grand, but it is no "Cannon" feel in that sample so i have to adjust the Low frequency, fall off and implement the sci-fi elemt, I created a lazer effect with the sample and combined that with the other mixed sample that had the "bass punch"
After the first iteration of the Mixed sample or in an earlier test with unmixed audio sample I set up the FMOD project with all the Events and parameters that I in hindsight believe we will have in the game, this so i can iterate later and remove excess or add small add ons if needed.
I start in the header to add enums.
eDescription will be used to bind Events wityh the GUID later, And eEventParameterName will be used to easier get the FMOD parameter strings.
For the eParameterName I add const char* member variables to write manually what the parameternames are named inside the FMOD project
I set up Bank* buffers and assign them to nullptr, however I do not use them more other than as "buffers" in the Event bindings
Here is the core essentials for this manager setup:
The AudioContext is a struct to contain the FMOD syetem API.
The Player is seperated from all other Game objects with its own 3D_ATTRIBUTES mainy for failsafe and to easier access that without searching for it later in containers.
myVolumeMixers is the first map that will hold all volume mixing, i bind the corresponding Buses with correct enum value.
myEvents is the bulk map that will hold in similar fashion created instaces paired with a gameobjects unique ID as a key.
myGlobal events is a copy in logic but seperated to be able to manage audio seperate from the game worlds 3D space.
myEventDescription is another map that will hold One event at dessignated eDescription enum Key with corresponding name.
myeventParameterName will be for accessing the Parameter string.
This is the struct tht holds the FMOD systems API.
In the Initialization function called Init all that follows in this chapter runs once there in startup.
I assign the strings for the parameternames first due to the manual implementation and to find it stright away for ease of use.
Next i Prepare the mixers and decription maps so there is no issues later with memory issues I ahve it set up like this for readability and to make it easy to implement new mixing channels and descriptions.
All enums are allocated to their part in the map with nullptr as a buffer to be replaced later
And before I start allocate anything I need to create and Initialize the FMOD API
I get the generated .bank files from the folder I build them to from the FMOD project, and here i also use thos Bank* "buffers" they will not be used other than here, the audio will not het loaded properly otherwise
And so in order next up is the Volume mixers, at each key i allocate corresponding Bus
And per default I assign each bus to be set to 1.0f wich is the max in this case.
I do volume mixing in the FMOD project so that no audio sample peaks above any other, but the player lower the buses as he/she pleases later in game.
And next is the perhaps one of the major important maps; the myEventDecription. This map pair the relevant Event to corresponding enum value, and this will be important later when creating instances, for if the gameobject can't know where it Event are in the map, the game object will not be able to find it. In my wrapper i have made it so that the game object basicly tell the wrapper who the object is via it's unique ID and after that It can request how it want to sound like via the description enum value.
we will look at how this works further down!
The last map will be so bind the Events parameter name with an enum value and the loaded const char*.
It may be alot of text for this, But it's all for readability and definition.
Lastly we set the myPlayerTransform to a default value.
This is the end of Init and all of this only runs once when the game start, there is never any need to reload anything in this Init function. everything is neatly packed on a well structured shelf for the user of this wrapper to use.
Next we look on some of the functions we utilize
For ease of use of the AudioManager I created an InitPlayer to not have any confusions and to make it modular.
The player still use MyEvents Map like all other game object.
I'll talk in the end about why PlayEvent is here.
(1)
This is perhaps the most important Function to explain;
the values we pass is WHAT, WHO and WHERE?
WHAT:
Is what are we? how do we want to sound like? in our example we want to sound like:
eDescription:: ePlayer,
WHO:
Is who we are, the player as a game object have an unique ID, perhaps 233 and that will be our key in myEvents.
WHERE:
Is were do we initially start with our audio, one can use any position but usually we just use our game objects position.
(2)
When we know what is requested we create a new instance and unless it's the player we create a new 3D_ATTRIBUTES aswell, and allocate it in a transform map for update later later.
After we create a new EventInstance and allocted positions we insert the newEvent into the myEvents map, however the new instnce have yet no clue what Event it want to use, So we use the myEventDecribtion at our requested enum key and thus we find the correct Event, with our describtion we create the instance with out newly empy EventInstance at our game objects key value. So now when we call the myEvents. at(anID) we can utilize all the functions of the specific Event!
The global events use the same logic as the previous function but without any position.
However considering that we don't have any ID system for things like music we are currently as for now to assign that hard coded. ill talk about this in the end.
Here are two functions that use similar logic, we just update the position, but considering the player needs more information bacause of the camera matrix i split the player out from the UpdatePosition, mainly because doing that we can skip going thou extra checks to see if it is the player or not and in the game code it is easier to find the player and everything else is default to be all other game objects.
Pretty stright forward:
getter and setter for the volume.
We ask for what volume mixer channel we want to use and update the volume value
Therse two work the same but likewise the player I have split these two apart but the reason is that they do not use the same map.
The setParameterByname only approves some kind of string and float.
But we show our ID key and then we use the function GetParameterName and use that to pass the requested string, but One can by design choose to type that manually if one wishes.
Then we pass a value of that parametername.
we can easily se the power of the structure here in how the myEvents find correct Event just by an ID. the user of this manager dont need to think about finding correct Event via this design choise.
we also for failsafe assure that the map have this ID and is not empty.
Here we can Easily play the game objects Event using the map myEvents.
we still do failsafe checks.
This function is to return the const char* we have allocated at corresponding enum value for the editParameter function.
And lastly we update the listeners position and finally we update the whole FMOD system always last.
and the game loop continue
Next we will look on how we use this manager in the game code
After we have run Init in the Game engine at start up, we can access the AudioManager from the game engine Instance
We GetAudioManager and access InitPlayer.
we fetch WHAT, WHO, WHERE.
DONE! all is allocated and we know where
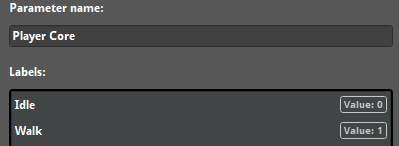
for less syntax, we do an auto and call it audio
we want to edit some parameters to the Event; we want to play walk and pause it
I call all basic player behaviour for "core" and in all paramets i use 0 as "mute".
We just change the parameter to 1 and we now have a walking audio for the player when the player is walking
Some more exaples of how we change the Event;
Here we look at how i manipulate the Event to play different audio for the weapon.
What the player do is Actions.
But i added "key" wich is a special case parameter SFX for the weapon trigger
Mail: kontakt@adamdahlin.se